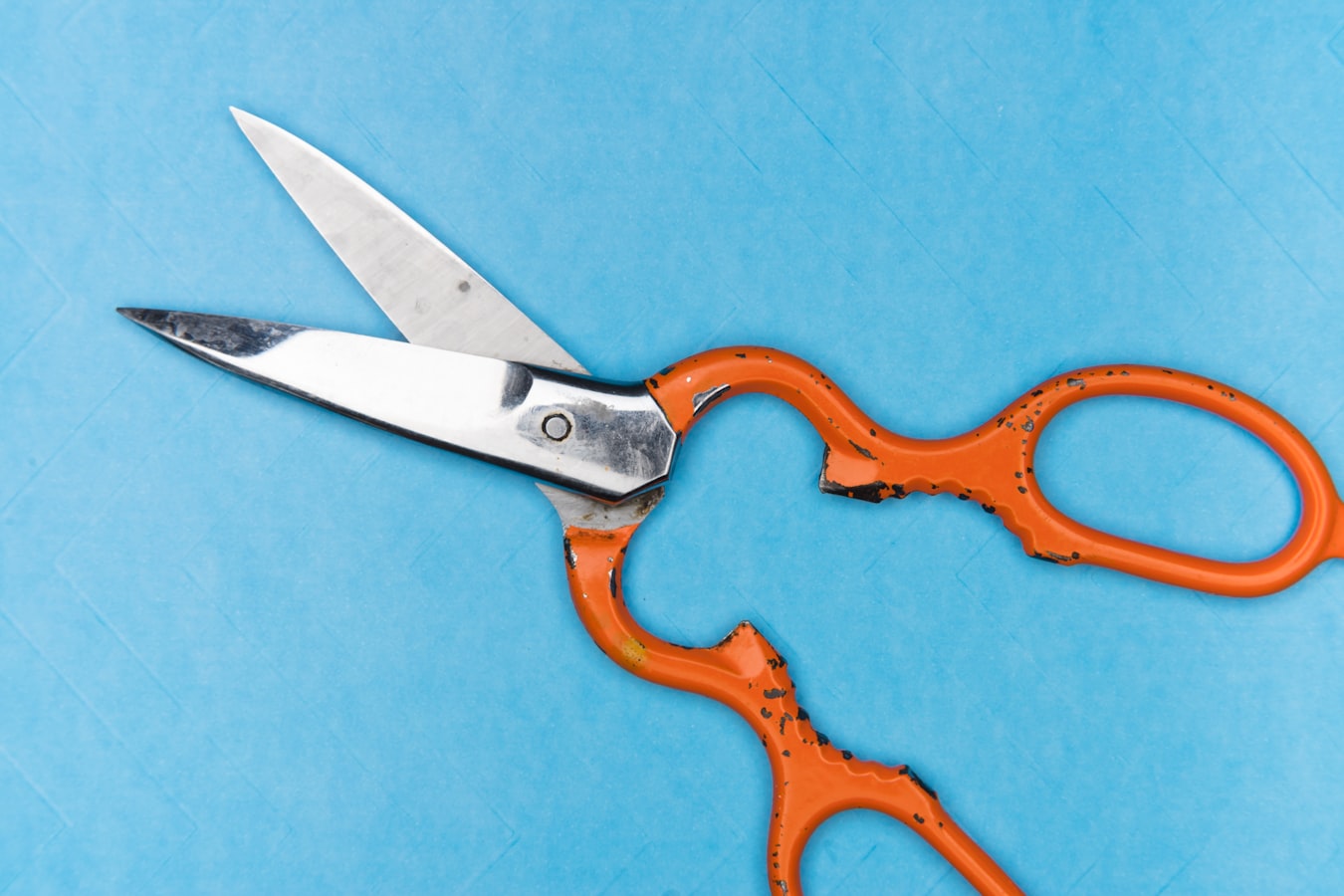
最近專案需求要把後端傳過來的字串切割成字串,並只 post 到後端只有數字的部份。
這次使用的套件為 element-ui 裡面的 tree 元件。
使用套件
情境如下:
- 會有一個樹狀圖。
- 需要有點選資料的 checkbox。
- 其中的文字是從後端 API 抓回來的資料。
- 文字前面的標籤用 v-if 做判斷,其中屬性 folder:true 是顯示
資料夾
, folder:false 則顯示標籤
。
畫面如下:

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| <el-tree :data="data" node-key="key" ref="tree" show-checkbox default-expand-all @check="getCheckedKeys" > <span class="custom-tree-node" slot-scope="{ data }"> <span> <i v-if="data.folder" class="far fa-folder-open"></i> <i v-else class="fas fa-tag"></i> {{ data.title }} </span> </span> </el-tree>
|
其中有使用到 tree 元件的方法,@check="getCheckedKeys"
,簡單說就是可以取得 checkbox 的值,但要搭配 show-checkbox
屬性才能使用。(詳細說明可以看官方文件)
引入套件
1 2 3 4
| import ElementUI from "element-ui"; import "element-ui/lib/theme-chalk/index.css"; const Vue = require("vue"); Vue.use(ElementUI);
|
如何切割字串
目標是要把回傳的資料格式做修改,回傳一開始是一個陣列,裡面有數個資料,切割使用 split 陣列方法,但只能使用在字串,目前資料為陣列,所以要取出來變成字串。
一開始先使用套件給予取值的方法,然後看看取出什麼。
1 2 3 4
| getCheckedKeys() { let dataKeys = this.$refs['tree'].getCheckedKeys(); console.log(dataKeys); },
|
是陣列,然後要先把他轉成字串。
1 2 3 4 5 6
| getCheckedKeys() { let dataKeys = this.$refs['tree'].getCheckedKeys(); console.log(dataKeys); let dataStr = dataKeys.toString(); console.log(dataStr); },
|
得到字串後,可以看到字串中間有一個底線,但後端只要數字的部份,所以要來做切割,並取出數字的部份。
1 2 3 4 5 6 7 8
| getCheckedKeys() { let dataKeys = this.$refs['tree'].getCheckedKeys(); console.log(dataKeys); let dataStr = dataKeys.toString(); console.log(dataStr); let dataSplited = dataStr.split("_").pop(1); console.log(dataSplited); },
|
確認可以正確切割後,但我有多個應該要用迴圈,另外如果要取陣列多個一定也需要使用迴圈來取。
提示:看到陣列就要想到使用迴圈。
所以我使用 forEach 來取,就會知道 item 取出來的已經是字串,真是太好了!
1 2 3 4 5 6
| getCheckedKeys() { let dataKeys = this.$refs['tree'].getCheckedKeys(); dataKeys.forEach((item) => { console.log(item); }); },
|
這樣就可以來做切割的動作,再把資料丟回 data 設定好的空陣列即可,然後這個功能就做完了(灑花)!
1 2 3 4 5 6 7
| getCheckedKeys() { let dataKeys = this.$refs['tree'].getCheckedKeys(); dataKeys.forEach((item) => { let tagId = item.split('_')[1]; this.tag_ids.push(tagId); }); },
|
.
.
.
.
.
.
.
當然事情絕對不會如前端菜雞想得一樣…
遇到問題
undefined 無法切割
前面提到 split 方法只能使用在字串,但後端資料設定資料夾部份的值是 undefined,所以在測試的時候, tag 的值是可以正確,而後端也只 type = 'tag'
的值,所以在改寫成這樣。
1 2 3 4 5 6 7 8 9 10 11 12
| getCheckedKeys() { let dataKeys = this.$refs['tree'].getCheckedKeys(); dataKeys.forEach((item) => { if (item !== undefined) { let keySplited = item.split('_')[0]; if (keySplited == 'tag') { let tagId = item.split('_')[1]; this.tag_ids.push(tagId); } } }); },
|
重複傳值
解決型別問題後,在測試的時候又遇到會重複傳值的問題,選擇 checkbox 後,出現一個現象,
會把之前的選項也列入空陣列中,例如有三個選項可以勾選,選到資料會變成 1 , 12, 123 結果變成六個選項…
後來觀察資料發現是陣列會一直保留前一次的所選的值,所以會不斷的添加,後來解決辦法。
把陣列清空後,再重新把值放進陣列。
1 2 3 4 5 6 7 8 9 10 11 12 13
| getCheckedKeys() { this.tag_ids = []; let dataKeys = this.$refs['tree'].getCheckedKeys(); dataKeys.forEach((item) => { if (item !== undefined) { let keySplited = item.split('_')[0]; if (keySplited == 'tag') { let tagId = item.split('_')[1]; this.tag_ids.push(tagId); } } }); },
|
這個功能才是正確完成了!
以此紀錄!
參考資料