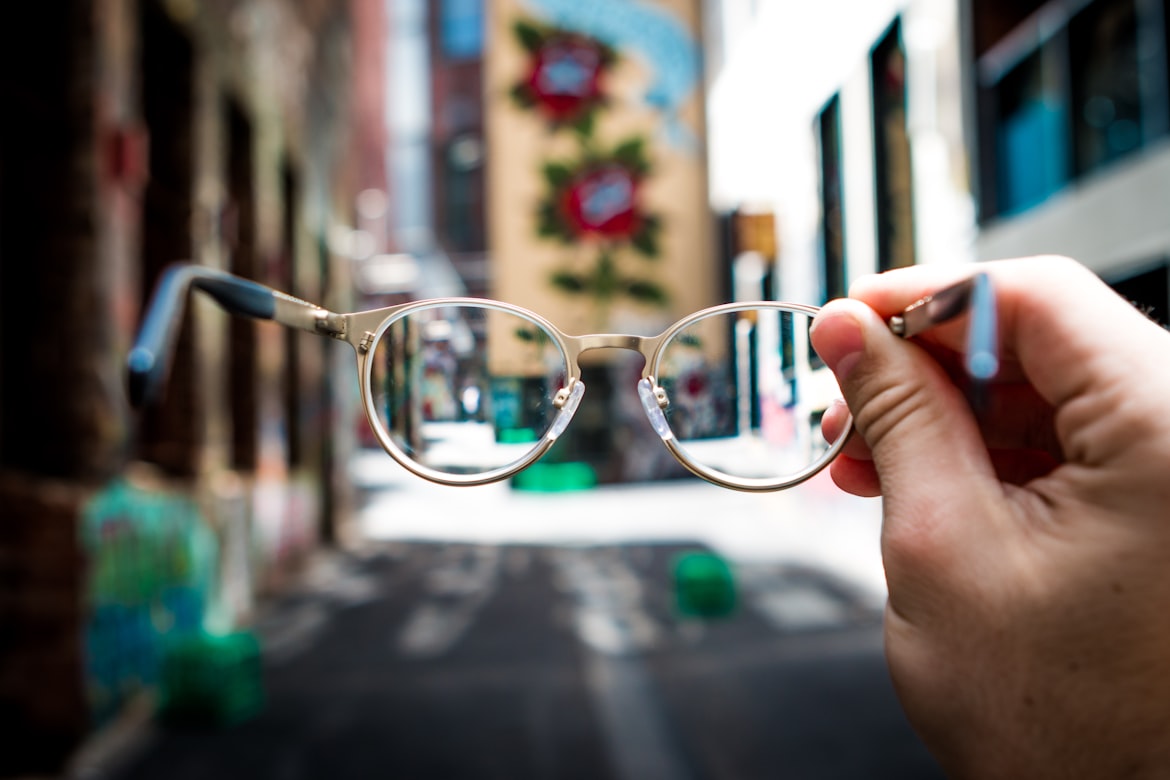
本篇記錄 Watch 基本觀念與使用方式,透過練習更知道如何應用。
什麼是 Watch
以下是官網介紹:
計算屬性允許我們聲明性地計算衍生值。然而在有些情況下,我們需要在狀態變化時執行一些“副作用”:例如更改 DOM,或是根據異步操作的結果去修改另一處的狀態。
在 Option API 中,我們可以使用 watch 選項在每次響應式屬性發生變化時觸發一個函數。
簡單來說就是監聽資料的監聽器,可以即時監聽資料變動的情況。
下方為簡易的範例:
基本用法
這邊使用一個 input,預期透過 watch 來看看是否有超過十個數字,若有,就回傳對應的文字,並使用 computed 渲染於畫面。
template
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| <div class="text-left w-96"> <h4 class="py-2 text-gray-500 underline"> <strong>基礎用法</strong>,監聽 number 變數,並存取在結果 </h4> <label> <span>輸入資料:</span> <input type="text" class="border border-gray-400 p-2 rounded-md focus:ring-4 outline-none" v-model="number" /> </label> <br /> <p>結果:{{ resultBasic }}</p> </div>
|
script
基本用法相當簡單:
- data 中透過 v-model 雙向綁定在模板上的變數區塊。
- 在 computed 寫入要判斷數字長度若大於等於 10,就要回傳對應的文字。
- 使用 watch 物件監聽資料,會使用要監聽的變數當作函式名稱,這邊一樣命名為 number,並有兩個參數,前面為新的值,後面為舊的值,參數名稱可自訂義,這邊統一命名為 newValue 與 oldValue,比較易讀且好理解。
- 當我在 input 輸入值時,watch 就會監聽到改變的值,當超過 10 個數字的時候便會出現對應的文字。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| <script> export default { name: "VueTailwindcssWatchPractice",
data() { return { number: 0, resultText: "", }; }, computed: { resultBasic() { if (this.number.length >= 10) { this.resultText = `數字 ${this.number} 長度大於 10 位數`; } else { this.resultText = `數字 ${this.number} 長度小於 10 位數`; } return this.resultText; }, }, watch: { number(newValue, oldValue) { this.number = newValue; }, }, }; </script>
|
監聽多個變數
也可以同時監聽多個變數,用法跟基礎用法雷同,但這次是直接監聽兩個變數。
template
雙向綁定兩個變數 product 跟 price。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| <div class="text-left w-96 my-5"> <h4 class="py-2 text-gray-500 underline"> 監聽多個<strong>變數</strong>,並使用 computed 呈現結果 </h4> <label> <span>項目:</span> <input type="text" class="border border-gray-400 p-2 rounded-md focus:ring-4 outline-none" v-model="product" /> </label> <br /> <label class="block my-3"> <span>價格:</span> <input type="text" class="border border-gray-400 p-2 rounded-md focus:ring-4 outline-none" v-model="price" /> </label> <br /> <p>結果:{{ resultMutiVariable }}</p> </div>
|
script
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| <script> export default { name: "VueTailwindcssWatchPractice",
data() { return { product: "品項", price: 0, result: "", }; }, computed: { resultMutiVariable() { if (this.price.length >= 5) { this.result = `今天買了 ${this.product},價格是 ${this.price},太貴了!!`; } else { this.result = `今天買了 ${this.product},價格是 ${this.price},好划算!!`; } return this.result; }, }, watch: { product(newValue, oldValue) { this.product = newValue; }, price(newValue, oldValue) { this.price = newValue; }, }, }; </script>
|
監聽物件資料
這個用法也常使用,但方便的是只要物件有更動資料,會直接抓取整包物件回來更新,就不用擔心會少掉欄位。
template
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| <div class="text-left w-96 my-5"> <h4 class="py-2 text-gray-500 underline"> 監聽<strong>物件資料</strong>,並使用 computed 呈現結果 </h4> <label> <span>項目:</span> <input type="text" class="border border-gray-400 p-2 rounded-md focus:ring-4 outline-none" v-model="products.name" /> </label> <br /> <label class="block my-3"> <span>價格:</span> <input type="text" class="border border-gray-400 p-2 rounded-md focus:ring-4 outline-none" v-model="products.price" /> </label> <br /> <p>結果:{{ objectResult }}</p> </div>
|
script
這邊監聽物件的方式會是深層監聽,只要物件有更新資料,便會透過 newValue 的參數傳入整包物件資料。固定格式如下:
- 一樣會用物件的名稱作為監聽物件名稱。
- 會用一個 handler 函式做為監聽,參數如前面所述。
- 並使用 deep 屬性為 true,代表要做深層監聽。
1 2 3 4 5 6 7 8 9
| watch: { products: { handler(newValue, oldValue) { }, deep: true, }, },
|
完成基本物件監聽起手式後,把對應的變數寫好,watch 監聽使用定義好的物件去接收整包更新後的值,再用 computed 渲染在畫面上。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| <script> export default { name: "VueTailwindcssWatchPractice",
data() { return { products: { name: "品項", price: 0, result: "", }, }; }, computed: { objectResult() { return (this.products.result = `今天買了 ${this.products.name},價格是 ${this.products.price}`); }, }, watch: { products: { handler(newValue, oldValue) { this.products = newValue; }, deep: true, }, }, }; </script>
|
以上就是 Watch 的基本使用方式以及基礎應用。
Demo: https://codepen.io/hnzxewqw/pen/BaqavoQ